Do you need a way to quickly display a HTML table of data from a JSON response, I got a solution. The key to doing all this is just a little JavaScript to parse your JSON results. By looping over the array of objects, you can build a dynamic table (no predefined columns) in just a few seconds. This solution can be used for any language, since I use .NET / jQuery most of my day, this is a ASP.NET demo.
If you just want to see a full working demo, download the sample project at the bottom of the article. It’s a VS 2008 project build-ready!
This user control was an enhancement to an existing user control whose core functionality is to take a user supplied T-SQL statement and generate a nicely formatted HTML table. The existing control has worked great for years, but since I recently moved my code from 2.0 to 3.5, I figured I’d give this module a little attention. I found pages using the module to sometimes take 15+ seconds to load because of slow database servers. I enabled caching to try and work around the problem, but users told me that some queries need to be real-time. The solution was to move from a standard GridView control to a AJAX jQuery solution that would pulled the data and convert the results to a pretty HTML table. I used Google to search for a existing solution, but everything I found used 3rd party components or required a results (defined columns) template (e.g. Name and Object[i].Name). Since my tool is designed for ad-hoc queries, I wanted the rendering logic to require no predefined facts about the data.
My demo has 3 external requirements, which are all FREE (jQuery, JavaScript JSON helper, JSON.NET).
Newtonsoft.Json.dll
jquery-1.3.2.debug.js
json.debug.js
In addition to these requirements, I added a few layout files to the demo to make the HTML table look nice. Nobody likes a ugly demo…
images / loading.gif
styles / default.css
The only thing left was the results page, rendering JS logic and the web service.
Here is the JavaScript function used to convert a JSON array of objects into an HTML table.
function CreateDynamicTable(objArray) {
var array = JSON.parse(objArray);
var str = '<table class="lightPro">';
str += '<tbody><tr>';
for (var index in array[0]) {
str += '<th scope="col">' + index + '</th>';
}
str += '</tr>';
str += '</tbody><tbody>';
for (var i = 0; i < array.length; i++) {
str += (i % 2 == 0) ? '<tr class="alt">' : '</tr><tr>';
for (var index in array[i]) {
str += '<td>' + array[i][index] + '</td>';
}
str += '</tr>';
}
str += '</tbody>'
str += '</table>';
return str;
}
Pretty simple, right… I pass in an object array that I iterate over to grab all the row/column values. I use the first row to grab the column names and place them inside the table header.
[Update 4/30/10] – I created 2 function that are improvements on this in a new posting. Check it out to see example use and download the JS code. Much better solution than hard-coding the values. Read Now…
Here is the actual page that makes web service call and returns displays the HTML table.
<script src="scripts/jquery-1.3.2.debug.js" type="text/javascript"></script>
<script src="scripts/json.htmTable.js" type="text/javascript"></script>
<script src="scripts/json.debug.js" type="text/javascript"></script>
<script type="text/javascript">
$(document).ready(function() {
$.ajax({
type: "POST",
url: "DemoSvc.asmx/GetJSONTableContents",
contentType: "application/json; charset=utf-8",
dataType: "json",
data: "{}",
success: function(res) {
$('#<%= DynamicGridLoading.ClientID %>').hide();
$('#<%= DynamicGrid.ClientID %>').append(CreateDynamicTable(res.d)).fadeIn();
}
});
});
</script>
<form id="form1">
<div id="DynamicGrid">
<div id="DynamicGridLoading">
<img src="images/loading.gif" alt="" /><span> Loading Data... </span></div>
</div>
</form>
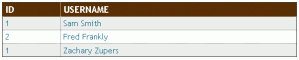
The code is pretty simple and there is no code behind, everything is done in the web service and injected via jQuery. The could have be done in a HTML page or any other language, nothing specific to ASP.NET right here.
The code for the web service is pretty basic, just be sure to look at the Service() method that is used to convert the DataTable to JSON. This is where the magic happens. This method is a wrapper to the JSON.NET library and chooses some default settings needed for the conversion.
In summary, this little switch removed the the blocking being caused by my slow SQL queries. My pages now instantly load **POOF**. During loading, they display a loading icon/text so the users know something is loading! Since caching was already being used for some queries, I change the code to cache the DataTable in the web service call.
VS2008 – ASP.NET DataTable to JSON to Dynamic HTML Table Demo