Over the past few weeks I’ve been trying to learn how WCF can be used for REST. I found lots of information, but I also found lots of broken and incomplete demonstrations. Being new to WCF/REST, I really needed a working demo to see what I was doing wrong in my setup. After using the mighty Google for a few days and reading a ton of blog posts, I was able to piece together a working solution. I then played for a few days to make sure I could consistently reproduce working code and decided to build a working sample to show how to use WCF/ASMX services with ASP.NET 3.5 SP1 and jQuery. This demo was designed to give people the basic instructions on what to setup to be able to send/receiving data from the browser using jQuery with the server.
Demo 1-4 shows you the ASMX and the WCF equivalents for doing the basics (Get String, Get Array, Send String, Send Array), and Demo 5 shows you how to use a WCF Data Contract with a Wrapped/Bare message format. To make things easier to see, I log all JSON requests/results to the web page for inspection. This also allows you to see how requests are non-blocking and can be received out of order depending on processing time.
Demo Screen Shot
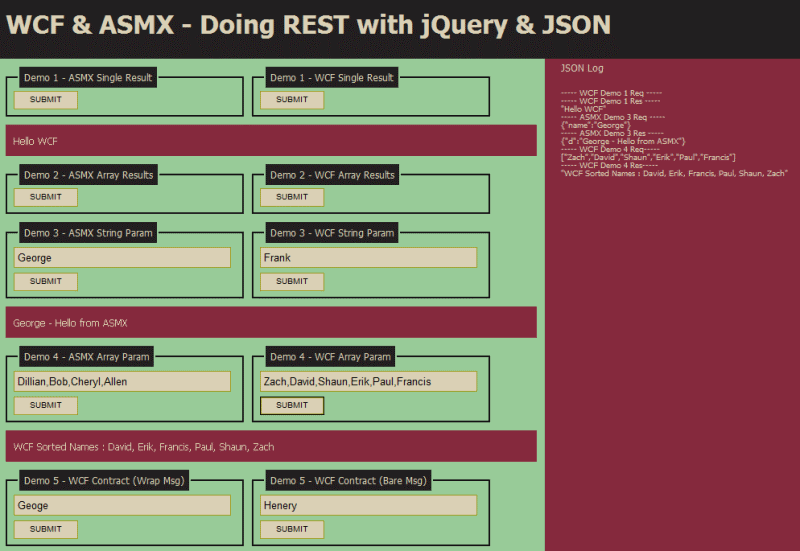
How to add a ASMX Web Service (ASP.NET Web Application w/.NET 3.5 SP1 Framework)
- Add New Item – Web Service
- Un-comment “[ScriptService]”
That’s it, your done. To access your web service via jQuery, use the following syntax.
$.ajax({
type: "POST",
url: "WebService1.ASMX/HelloWorld",
contentType: "application/json; charset=utf-8",
dataType: "json",
data: "{}",
success: function(res) {
// Do your work here.
// Remember, the results for a ASMX Web Service are wrapped
// within the key "d" by default. e.g. {"d" : "Hello World"}
}
});
How to add a WCF Service (ASP.NET Web Applicaiton w/.NET 3.5 SP1 Framework)
- Add New Item – WCF Service
- Right-click on the service and choose “View Markup”.
- Add the following line to the ServiceHost declaration: Factory=”System.ServiceModel.Activation.WebServiceHostFactory”
- Add a reference to the project for “System.ServiceModel.Web”.
- Open the interface associated with the service (e.g. IService1.cs) and add the following using statement “using System.ServiceModel.Web;”.
- Inside the interface, set the attributes on the method to support JSON & POST or GET request types.
-
[OperationContract] [WebInvoke( Method = "POST", ResponseFormat = WebMessageFormat.Json)]
- Open the web.config and comment out the following lines:
<!--<behaviors>
<endpointBehaviors>
<behavior name="Web.Service1AspNetAjaxBehavior">
<enableWebScript />
</behavior>
</endpointBehaviors>
</behaviors>
<serviceHostingEnvironment aspNetCompatibilityEnabled="true" />
<services>
<service name="Web.Service1">
<endpoint address="" behaviorConfiguration="Web.Service1AspNetAjaxBehavior"
binding="webHttpBinding" contract="Web.Service1" />
</service>
</services>-->
That’s it, your done. To access your web service via jQuery, use the following syntax.
$.ajax({
type: "POST",
url: "Service1.svc/DoWork",
contentType: "application/json",
dataType: "json",
success: function(res) {
// Do you work here.
}
});
I found a lot of blog posts pointing to WCF Service Factory projects, which require a completely different setup. The sample above is for adding a WCF Service to a Web Application.
While debugging this, I used Firebug for Firefox and Fiddler to see the ajax & JSON data fired between the client and server. When you use fiddler to debug a local website, you need to add a “.” after the domain name to cause fiddler to catch the traffic (e.g. http://localhost.:1234/WebSite1). Hopefully the demo compiles and runs without problems, so you can see a quick 1-2-3 on how to get WCF up and running quickly!
I’m by far no expert on these technologies, so if anybody has suggestions on what I could be to make this demo better… Let me know! Hopefully seeing a working demo will help somebody else get a jump start on using jQuery to consume REST services.
#1 by jason robertson on April 25, 2011 - 7:17 pm
Very Help full, surprised there are no other comments on this
#2 by Zach on April 26, 2011 - 3:15 pm
Thanks, glad it was helpful…